Build a Basic Frontend
Building the Frontend
Let's build a basic frontend, to acquaint you with the native client SDK(s).
While this is a basic example, it'll give you a taste of what to expect from building a proper frontend for Parlant.
Start by creating the client project under the tutorial directory:
$ cd parlant-tutorial # If you're not already in it
- Python
- TypeScript
$ poetry new basic-client
$ cd basic-client
$ poetry add parlant-client
$ touch client.py
$ mkdir basic-client
$ cd basic-client
$ deno install npm:parlant-client
$ touch client.ts
Now let's start adding some code. First, we'll initialize the ParlantClient
object.
- Python
- TypeScript
from parlant.client import ParlantClient
client = ParlantClient(base_url=SERVER_ADDRESS)
import { ParlantClient } from 'parlant-client';
const client = new ParlantClient({ environment: SERVER_ADDRESS });
Note: For SERVER_ADDRESS
, if running locally, replace with http://localhost:8800
.
Load Your Agent
Load Mr. Bitman using the agent ID you have from before. If you lost it, you can find again it by running $ parlant agent list
.
- Python
- TypeScript
AGENT_ID = "<your-agent-id>"
agent = client.agents.retrieve(AGENT_ID)
const AGENT_ID = "<your-agent-id>";
const agent = await client.agents.retrieve(AGENT_ID);
Create a Session
Now, let's start a new interaction session with our agent.
- Python
- TypeScript
session = client.sessions.create(agent_id=AGENT_ID, allow_greeting=False)
const session = await client.sessions.create({
agentId: AGENT_ID,
allowGreeting: false,
});
For this simple use case, you can see that we're not allowing the agent to greet the customer proactively. Otherwise—depending on the guidelines installed and the customer they're talking to—agents in Parlant can proactively initiate a chat with a customer. This proactivity feature of Parlant agents comes in handy in certain real-life service scenarios. Keep it in mind!
Let's build our chat loop. See if you can notice something peculiar about how it works!
- Python
- TypeScript
while True:
customer_message = input("Customer: ")
if customer_message in ("quit", "bye", "exit", "cya l8r m8"):
break
customer_event = client.sessions.create_event(
session_id=session.id,
source="customer",
kind="message",
message=customer_message,
)
agent_event, *_ = client.sessions.list_events(
session_id=session.id,
source="ai_agent",
kinds="message",
min_offset=customer_event.offset,
)
# Let type-checker know we do have data here
assert agent_event.data
agent_message = agent_event.data["message"]
print(f"Agent: {agent_message}")
while (true) {
const customerMessage = prompt("Customer: ") || "";
if (["quit", "bye", "exit", "cya l8r m8"].includes(customerMessage)) {
break;
}
const customerEvent = await client.sessions.createEvent(session.id, {
source: "customer",
kind: "message",
message: customerMessage,
});
const [agentEvent] = await client.sessions.listEvents(session.id, {
source: "ai_agent",
kinds: "message",
minOffset: customerEvent.offset,
});
const { message } = agentEvent.data as { message: string };
console.log(`${agent.name}: ${message}`);
}
Unlike low-level LLM completion APIs, where each request (or prompt) is immediately answered with a response (or completion), with Parlant agents the communication model is event-driven rather than completion-based.
This means that customers can always send messages (and even split what they want to say into several messages, as people often do in real life chat), and agents are always free to respond in their own time—just like a person on the other end of the line.
Running the Client
Ready to chat with Chip? Go ahead!
- Python
- TypeScript
$ poetry run python client.py
$ deno client.ts
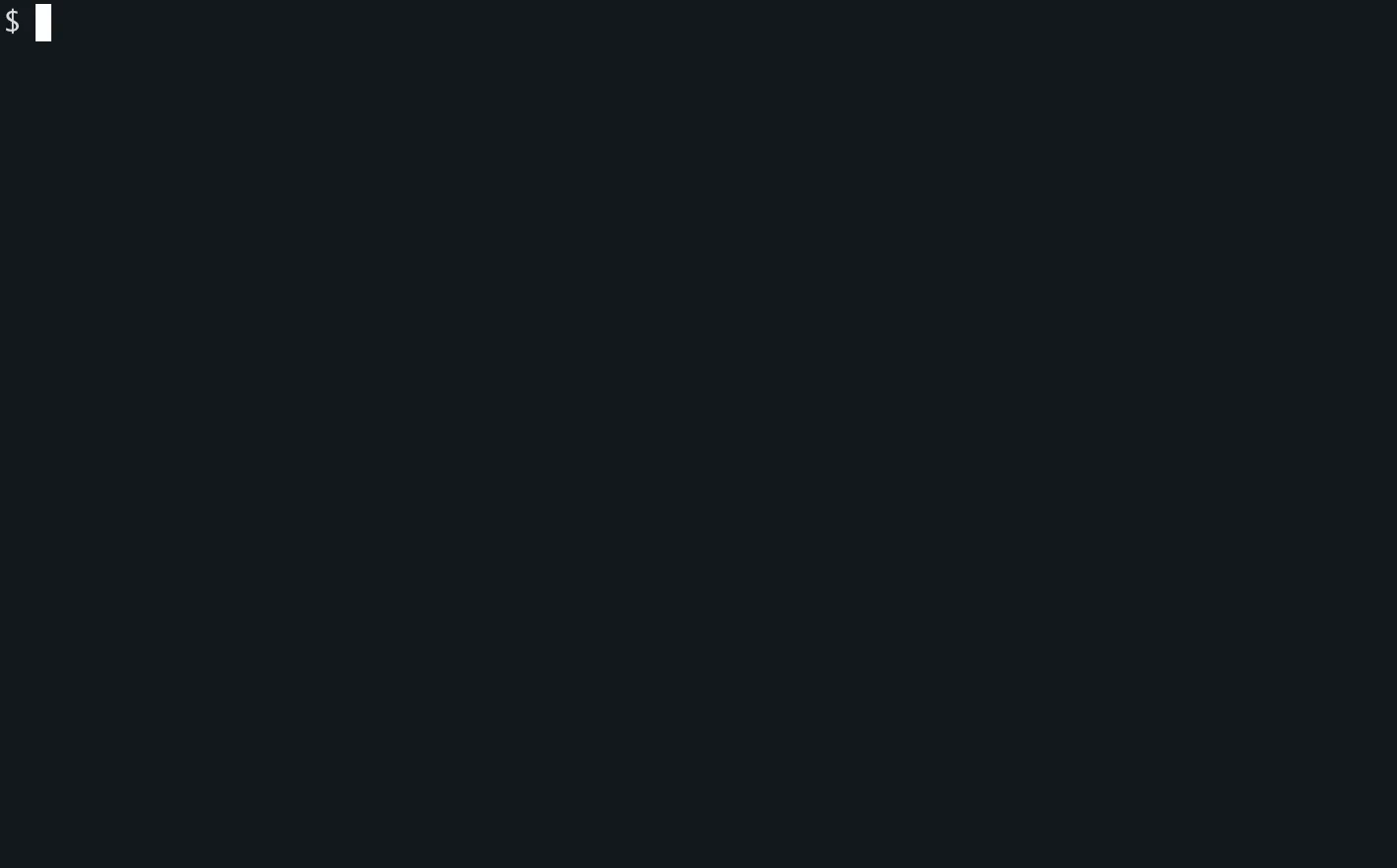
Recapping our frontend code, this is the logic we've written:
- Get a handle to our specific agent
- Create a new session to interact with it
- (In a loop)
- Append a new customer message
- Wait for the agent to generate a message, and fetch it
- Print the agent's reply message
Just FYI, there's a lot more you can do with sessions to create a truly responsive, sleek chat experience—such as indicating to the customer when the agent has read their last message, or currently typing out a reply. For more information, review the Sessions page.
Finally, an explanation for each little thingy in what we just wrote.
Code Breakdown
Thingy | Description |
---|---|
customer_message | The customer's message |
customer_event | The event created from the customer's message |
session_id | The ID of the session we created |
kind | The event type, set to "message" |
source | The origin of the event, which is "customer" . |
message | Contains the customer’s input. |
agent_event | Represents the response events received from the server. |
session_id | Selects events from our specific session ID |
kinds | Selects events by type, here set to "message" |
source | Selects events originating from the "ai_agent" |
min_offset | Ensures only events occurring after the last customer event (referenced by customer_event.offset ) are retrieved, capturing the agent's immediate response. |